Regular Expressions (Regex)
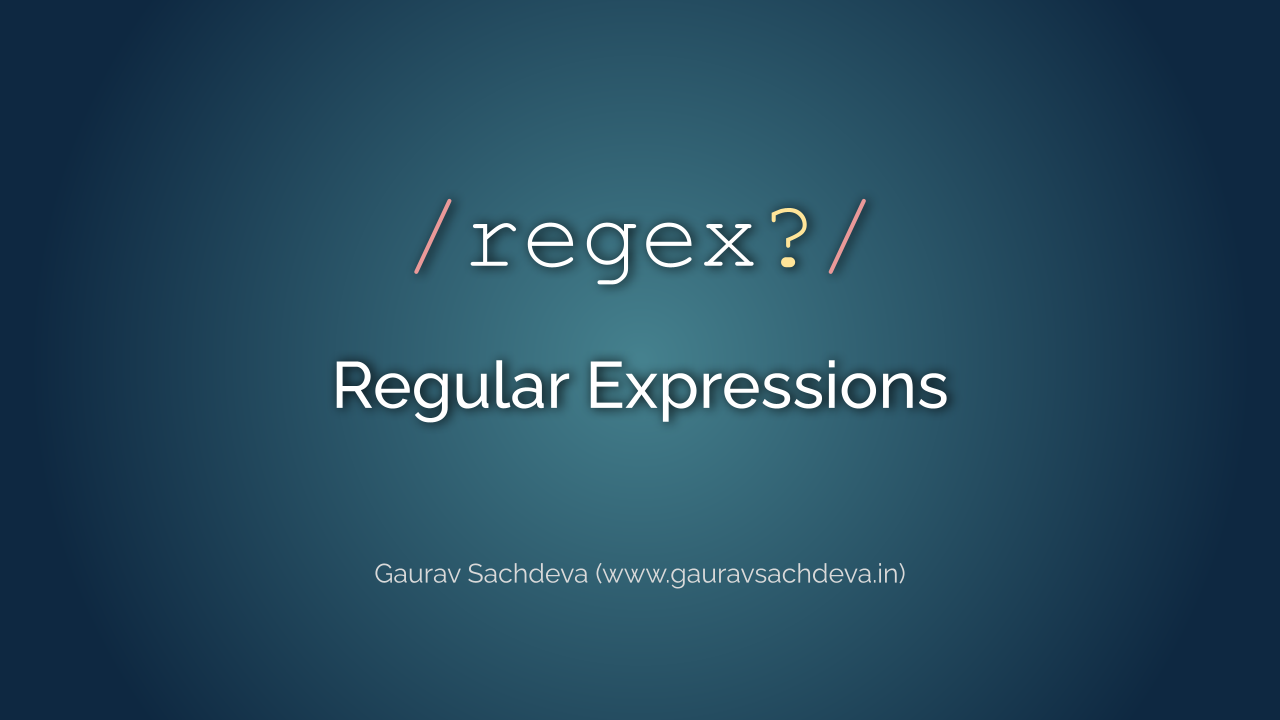
Regular Expressions, commonly known as Regex, are powerful tools for searching and manipulating strings. Whether you're validating input, searching for patterns in text, or extracting data, mastering regex can significantly enhance your coding efficiency. In this article, we'll dive into the essentials of regex, how it works, and practical examples to get you started.
What is Regex?
Regex is a sequence of characters that forms a search pattern. These patterns are used by string searching algorithms for "find" or "find and replace" operations on strings, or for input validation. Regex is supported in various programming languages, including JavaScript, Python, PHP, and many others.
Regex Syntax Basics
Regex is composed of literal characters and special metacharacters that have specific meanings. Here are some key components:
1. Literal Characters
Literal characters match themselves exactly. For example, the regex pattern hello
will match the string "hello" anywhere it appears.
2. Metacharacters
Metacharacters are characters with special meanings in regex. Here are some of the most common ones:
.
: Matches any single character except a newline.^
: Matches the start of a string.$
: Matches the end of a string.*
: Matches 0 or more repetitions of the preceding character.+
: Matches 1 or more repetitions of the preceding character.?
: Matches 0 or 1 occurrence of the preceding character.[]
: Matches any one character within the brackets.|
: Acts as an OR operator between different patterns.\
: Escapes a metacharacter to treat it as a literal.
3. Character Classes
Character classes allow you to define a set of characters to match:
[abc]
: Matches any one of the characters a, b, or c.[^abc]
: Matches any character except a, b, or c.[a-z]
: Matches any character in the range a to z.
4. Quantifiers
Quantifiers specify the number of times a character or group should be matched:
{n}
: Matches exactly n occurrences.{n,}
: Matches n or more occurrences.{n,m}
: Matches between n and m occurrences.
5. Groups and Capturing
You can group parts of your regex using parentheses, which also captures the matched content for later use:
(abc)
: Matches and captures "abc".(?:abc)
: Matches "abc" but does not capture it.
6. Anchors
Anchors are used to specify positions within a string:
^
: Start of a string.$
: End of a string.\b
: Word boundary.\B
: Non-word boundary.
Tips for Using Regex
- Test Regularly: Use online tools like regex101, regexr (my favorite) to test your regex patterns.
- Start Simple: Begin with a simple pattern and gradually add complexity.
- Document Your Patterns: Regex can be hard to read, so comment your patterns or use verbose mode in languages that support it.
- Watch Out for Performance: Complex regex patterns can be slow, especially with large datasets. Optimize your patterns and test performance.
Conclusion
Regular Expressions are an essential skill for any developer working with text. Although they can be intimidating at first, understanding the basics and practicing with real-world examples can make regex an incredibly useful tool in your coding arsenal. Whether you're validating input, searching through logs, or manipulating text, regex can save you time and effort.
Happy coding!