Using then() vs async/await in JavaScript
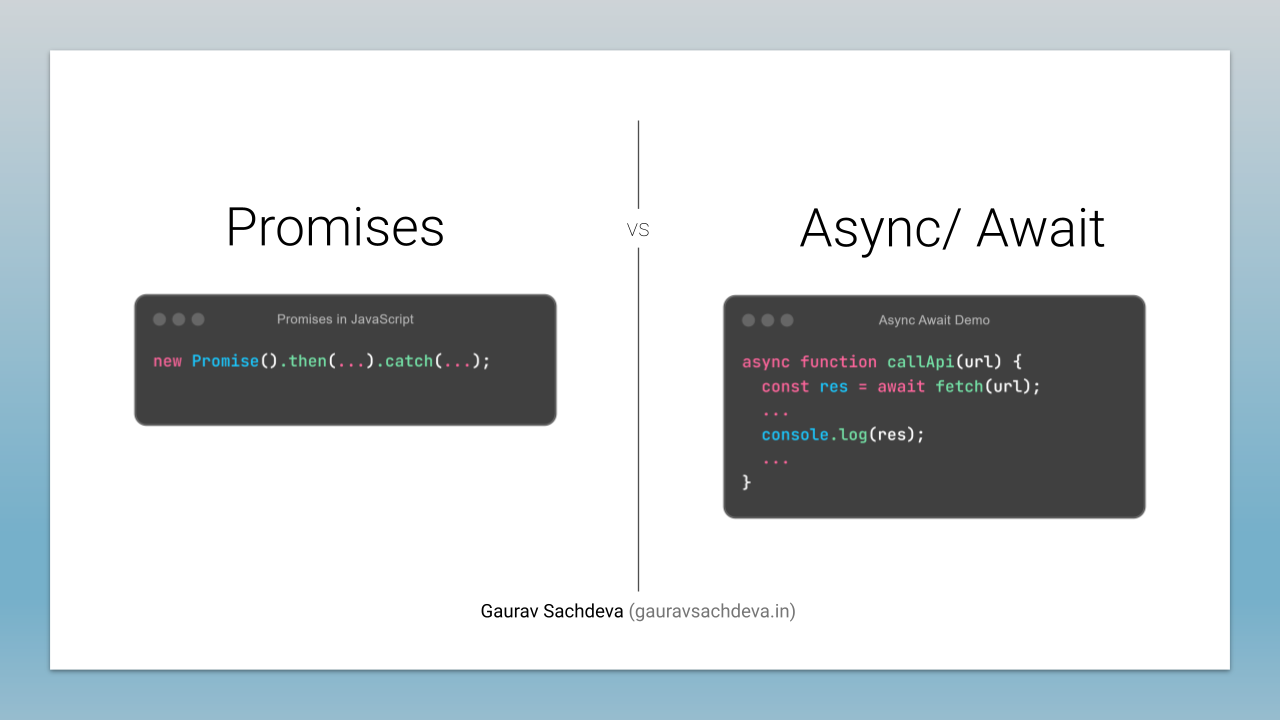
JavaScript's asynchronous nature is one of its most powerful features, allowing developers to handle operations like network requests, file reading, and timers without blocking the main thread. Promises and the async/await
syntax are two key tools for managing asynchronous code. In this article, we'll explore how to use then()
and async/await
, their differences, and when to use each.
Understanding Promises
A Promise is an object representing the eventual completion or failure of an asynchronous operation. It can be in one of three states:
- Pending: The initial state, neither fulfilled nor rejected.
- Fulfilled: The operation completed successfully.
- Rejected: The operation failed.
Using then()
The then()
method is used to handle fulfilled promises. It takes two arguments: a callback function for when the promise is resolved and another for when it is rejected.
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
In this example, fetch()
returns a promise. The first then()
processes the response, converting it to JSON. The second then()
logs the data. The catch()
handles any errors.
Using async/await
The async/await
syntax is a more recent addition to JavaScript, introduced in ES2017 (ES8). It allows you to write asynchronous code that looks and behaves more like synchronous code.
An async
function returns a promise, and within an async
function, you can use the await
keyword before a promise to pause execution until the promise is resolved.
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchData();
Here, fetchData
is an async
function. The await
keyword pauses the execution until fetch
and response.json()
are resolved, making the code easier to read and write.
Key Differences
- Readability:
then()
: Can become cumbersome with nested callbacks (callback hell).async/await
: More readable and closer to synchronous code.
- Error Handling:
then()
: Usescatch()
to handle errors.async/await
: Usestry/catch
blocks for error handling, making it consistent with synchronous code.
- Debugging:
then()
: Stack traces can be harder to follow due to nested functions.async/await
: Easier to debug with cleaner stack traces.
- Syntax:
then()
: Function chaining.async/await
: Synchronous-looking syntax for asynchronous operations.
When to Use then()
- When you are already working with promise-based APIs and need simple promise handling.
- When using libraries or codebases that predominantly use promises.
- When you need to handle multiple asynchronous operations that depend on each other.
When to Use async/await
- For better readability and maintainability, especially with complex asynchronous code.
- When you need to handle asynchronous operations in a more synchronous manner.
- For better error handling using
try/catch
.
Conclusion
Both then()
and async/await
have their places in modern JavaScript development. While then()
is suitable for simpler or already promise-based code, async/await
shines in scenarios requiring readable, maintainable, and debuggable asynchronous code. Understanding both approaches will help you choose the best tool for your specific needs, leading to cleaner and more efficient code.
Happy coding!
Bla bla